Qt Slot Sender
Introduction
Qt Network provide tools to easily use many network protocols in your application.
- @jsulm said in How to find sender in slot?: @cerr This is the answer: 'This class was mostly useful before lambda functions could be used as slots.' :-) Yup, I've figured this out by now as well. I'm still new to Lambda and am learning as I go.! Use lambda functions as you already do. 'I need to diustinguish them by grp' - what is grp?
- 当某一个Object emit一个signal的时候,它就是一个sender,系统会记录下当前是谁emit出这个signal的, 所以你在对应的slot里就可以通过 sender得到当前是谁invoke了你的slot,对应的是QObject-d-sender.
The most requested next C app you guys asked for was a calculator, so here it is. We’ll make a C GUI Calculator app in one video. Along the way we’ll learn a lot! We’ll cover a topic which confuses many people which is how to setup event handling with Signals and Slots.
TCP Client
To create a TCP connection in Qt, we will use QTcpSocket. First, we need to connect with connectToHost
.
So for example, to connect to a local tcp serveur: _socket.connectToHost(QHostAddress('127.0.0.1'), 4242);
Then, if we need to read datas from the server, we need to connect the signal readyRead with a slot. Like that:
and finally, we can read the datas like that:
To write datas, you can use the write(QByteArray)
method:
So a basic TCP Client can look like that:
main.cpp:
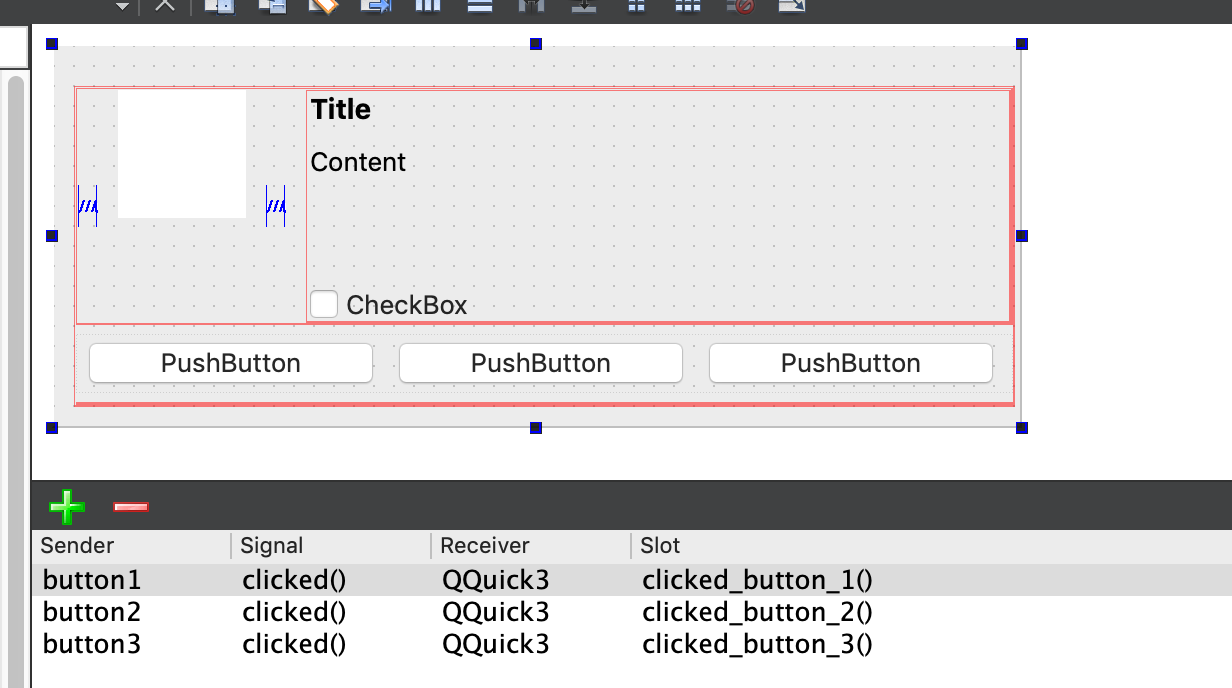
mainwindow.h:
mainwindow.cpp:
mainwindow.ui: (empty here)
TCP Server
Create a TCP server in Qt is also very easy, indeed, the class QTcpServer already provide all we need to do the server.
First, we need to listen to any ip, a random port and do something when a client is connected. like that:
Then, When here is a new connection, we can add it to the client list and prepare to read/write on the socket. Like that:
The stateChanged(QAbstractSocket::SocketState)
allow us to remove the socket to our list when the client is disconnected.
So here a basic chat server:
main.cpp:
mainwindow.h:
mainwindow.cpp:
(use the same mainwindow.ui that the previous example)
This tutorial is an introduction to Qt programming and will teach you how to create an application with Qt and C++. Full project and source code are provided.
Qt
Qt is a cross-platform framework and a collection of tools for creating applications which can run on desktop, embedded and mobile operating systems.
If you need to create an application with a GUI in C++ you should definitely consider Qt for it.
A simple example application
This tutorial will guide you through the creation of a basic window with a menu bar, a menu and a status bar. The final result while look like this:
Nothing too fancy, but these are the basic elements of most desktop applications and you can use this code as starting template for your projects.
When working with Qt, especially when developing cross-platform software, it’s recommended to use Qt Creator as IDE. The main reason is that Qt Creator is the official Qt IDE and it offers full integration with the framework and all the other development tools. Using other IDEs is possible, but I don’t recommend it and for this tutorial I will use Qt Creator.
Creating a new Qt project
The first step is to create a new project in Qt Creator from one of the available templates:
For a graphical application you want to select the Qt Widgets Application template.
The second step is selecting a name for your project and a location where to save it. For my project I decided to use “BasicApplication” for the name, but obviously you can use anything you want.
The third step is selecting the kit(s) for building your application.
Qt Creator kits are groups of settings used for building and running projects for different platforms.
Qt Slot Sender Cast
I am developing on Linux so I am going for the kit Desktop Qt 5.7.0 GCC 64bit, but you might see different kits if developing on/for different platforms.
The fourth step is defining the details of the initial main window class that will be generated by the template.
You can leave everything as it is, but make sure to uncheck the Generate form field. The form file is used by the integrated visual interface editor Qt Designer. We won’t need it because we will be coding the GUI from scratch.
In the final step of the project creation you will get a summary of what defined in the previous steps.
Here it’s also possible to set a version control system to use for the project, but feel free to not set any for now.
Qt Signal And Slots
Clicking on the button Finish will create the project and generate 3 C++ files (main.cpp, MainWindow.h and MainWindow.cpp) and a project file used by Qt Creator and qmake (the Qt building tool) to build the project.
The main
The main function initialises the application, then creates and opens the main window:
The last line of code launches the Qt application and waits for it to return an exit code before terminating the program.
The main window class
The main window is defined in the header file MainWindow.h and it inherits from QMainWindow:
It’s important to include the Q_OBJECT macro in all the classes that are inheriting from QObject.
In this simple example the constructor is the only public function:
Finally some private slots are declared:
Slots are handlers used to do something when an event happens. They will be explained in more detail later.
The main window implementation
All the MainWindow methods, including the slots, are implemented in MainWindow.cpp.
Qt Signal Sender
Most of the code will be placed in the constructor because it’s there where the elements of the window are created.
Window settings
We can start with some basic settings:
The function setWindowTitle sets the title of the window, whereas setMinimumSize sets the minimum size allowed when resizing it.
Adding a menu bar
Most applications have a menu bar with menus. Creating one in Qt is pretty simple:
Once the menu bar is created you need to set it for the main window calling the setMenuBar function.
After creating and setting the menu bar it’s time to create a first menu:
The “&” before “File” will enable the keyboard shortcut ALT+F to open the menu.
Now that we have a menu we can populate it with entries, which are QAction objects:
As you can see creating an entry and adding it to a menu is pretty straightforward.
What’s more interesting is connecting menu entries to functions so that something happens when they are clicked. This is achieved using the Qt function connect which in its standard format takes 4 parameters:
- sender – the element generating an event
- signal – the event generated by the sender
- receiver – the element receiving the event
- slot – the handler to execute when an event is received
In the case of the first menu entry we have the following scenario

- sender : action (the menu entry)
- signal : &QAction::triggered (a menu entry has been clicked)
- receiver : this (the event is handled by the MainWindow)
- slot : &MainWindow::OnFileNew (when the menu entry is clicked this function is called)
When building a menu it’s also possible to add a horizontal line to to separate logic blocks. It only takes one line of code:
The final entry of the File menu is going to be one to exit from the application:
This entry is connected to the function close defined in QMainWindow which, as expected, will close the window when the menu entry is clicked.
Adding a status bar
A status bar is useful to show messages to the user. Creating one is pretty simple:
As for the menu bar, it’s important to remember to set the active status bar calling the setStatusBar function.
Implementing the slots
In this simple example all the slot functions do is setting a message to show in the status bar like in the following code:
Obviously this is just an example and a real application will do something more useful with them.
Adding content to the window
Once the basic elements of the window have been created it’s time to add some content.
This is done by calling the function setCentralWidget and passing it a QWidget which contains the graphic elements the application needs to show and handle.
I decided to not add any central widget in my code to keep this example as simple as possible, but obviously you’ll need to do that in your application.
Source code
The full source code of this tutorial is available on GitHub and released under the Unlicense license.
References
All the Qt widgets discussed in this tutorial are documented in detail in the Qt Widgets C++ Classes section of the Qt documentation.
To learn more about Qt Creator check out the online manual.
Conclusion
Qt Connect Signal Slot
This was a simple tutorial to teach you how to create an application with Qt and C++. If you have any question about the code explained here feel free to leave a comment.
If you found it helpful please feel free to share it on social media using the share buttons below.
Subscribe
Don’t forget to subscribe to the blog newsletter to get notified of future posts.
You can also get updates following me on Google+, LinkedIn and Twitter.